Complexity (State Space)
Contents
Complexity (State Space)¶
This notebook covers Complexity measures that work in state space, including:
Lyapunov Exponent
# Setup notebook state
from nbutils import setup_notebook; setup_notebook()
from neurodsp.sim import sim_powerlaw, sim_synaptic_current, sim_combined
from neurodsp.utils import set_random_seed
# Import custom project code
from apm.io import APMDB
from apm.run import run_sims, run_sims_load
from apm.methods import lyapunov
from apm.methods.settings import LY_PARAMS
from apm.plts.sims import plot_ap_sims, plot_pe_sims, plot_sims_two
from apm.plts.settings import COLORS
from apm.plts.utils import figsaver
from apm.sim.examples import get_examples, check_examples
from apm.sim.settings import N_SIMS2 as N_SIMS
from apm.sim.defs import SIM_ITERS
Settings¶
# Get current sampling rate
fs = SIM_ITERS.fs
# Settings for saving figures
SAVE_FIG = True
FIGPATH = APMDB().figs_path / '24b_complexity_state'
# Create helper function to manage figsaver settings
fsaver = figsaver(SAVE_FIG, FIGPATH)
# Set the random seed
set_random_seed(111)
# Define collection of example signals
examples = get_examples()
Lyapunov Exponent¶
The Lyapunov exponent is a measure of measure defined for dynamical systems, which measures the rate of separation of trajectories in phase space.
In the context of EEG analyses, it is typically considered as a measure of complexity.
# Check the Lyapunov exponent values across some example signals
check_examples(examples, lyapunov, LY_PARAMS, 'lyapunov exponent')
Computed lyapunov exponent:
powerlaw : 0.0800
synaptic : 0.0780
knee : 0.0853
oscillation : 0.0000
burst : 0.6224
combined : 0.0835
comb_burst : 0.0823
comb_peak : 0.0832
Aperiodic Variations¶
# Run simulations calculating Lyapunov exponent across exponents
ly_sims_exp = run_sims(sim_powerlaw, SIM_ITERS['ap_exp'], lyapunov, LY_PARAMS, N_SIMS)
# Run simulations calculating Lyapunov exponent across exponents with an oscillation
ly_sims_comb = run_sims(sim_combined, SIM_ITERS['comb_exp'], lyapunov, LY_PARAMS, N_SIMS)
# Plot Lyapunov exponent estimates across aperiodic variations
plot_ap_sims(ly_sims_exp, ly_sims_comb, 'Lyapunov Exponent', **fsaver('ly_ap'))
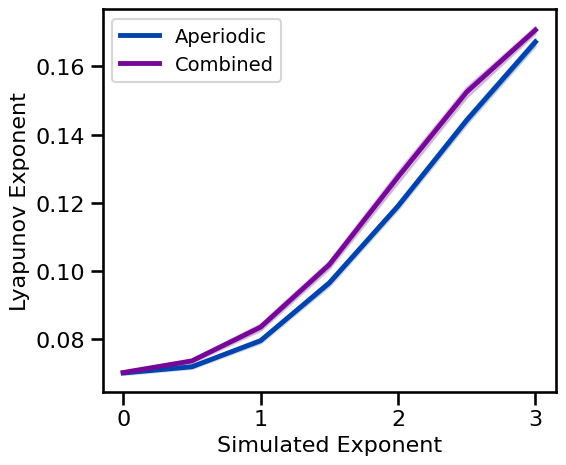
Periodic Variations¶
# Run simulations calculating Lyapunov exponent across oscillation frequency
ly_sims_freq = run_sims(sim_combined, SIM_ITERS['osc_freq'], lyapunov, LY_PARAMS, N_SIMS)
# Run simulations calculating Lyapunov exponent across oscillation power
ly_sims_pow = run_sims(sim_combined, SIM_ITERS['osc_pow'], lyapunov, LY_PARAMS, N_SIMS)
# Plot Lyapunov exponent estimates across periodic variations
plot_pe_sims(ly_sims_freq, ly_sims_pow, 'Lyapunov Exponent', **fsaver('ly_pe'))
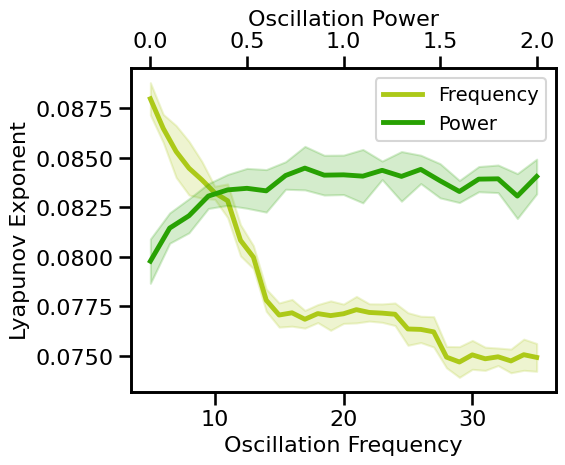
Knee Variations¶
# Run simulations calculating Lyapunov exponent across different timescales
ly_sims_tscales = run_sims(sim_synaptic_current, SIM_ITERS['syn_tscales'],
lyapunov, LY_PARAMS, N_SIMS)
# Run simulations calculating Lyapunov exponent across peak bandwidths (sims from file)
ly_sims_knee = run_sims_load('ap-knee-' + str(fs), lyapunov, LY_PARAMS, N_SIMS)
# Plot the estimated Lyapunov exponent across different timescales & knees
plot_sims_two(SIM_ITERS['syn_tscales'].values, ly_sims_tscales, 'Timescale',
SIM_ITERS['kn_knee'].values, ly_sims_knee, 'Knee Parameter',
ylabel='Lyapunov Exponent', color=COLORS['KN'], **fsaver('ly_kn_vars'))
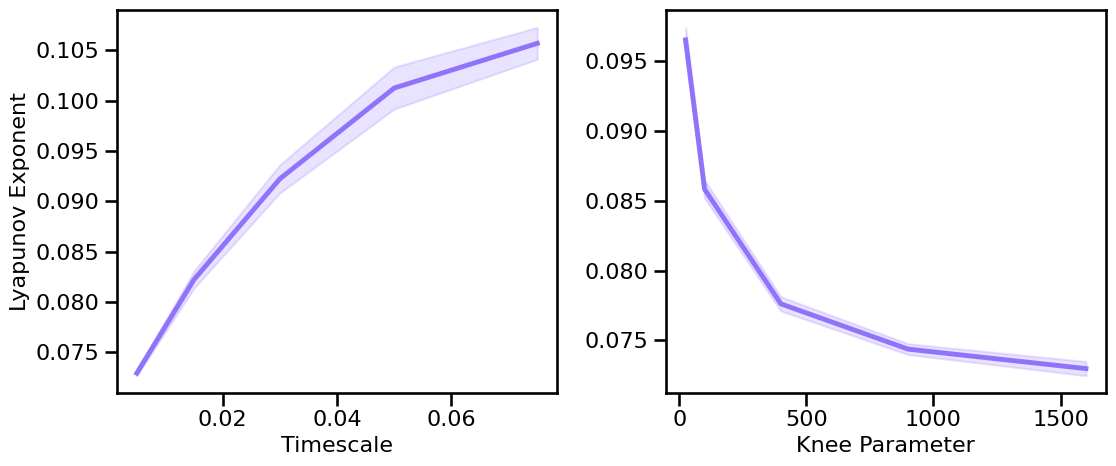
Peak Variations¶
# Run simulations calculating Lyapunov exponent across peak bandwidth (sims from file)
ly_sims_bw = run_sims_load('comb-bw-' + str(fs), lyapunov, LY_PARAMS, N_SIMS)
# Run simulations calculating Lyapunov exponent across burst probabilities
ly_sims_burst = run_sims(sim_combined, SIM_ITERS['comb_burst'], lyapunov, LY_PARAMS, N_SIMS)
# Plot the estimated Lyapunov exponent across periodic variations
plot_sims_two(SIM_ITERS['peak_bw'].values, ly_sims_bw, 'Bandwidth',
SIM_ITERS['comb_burst'].values, ly_sims_burst, 'Burst Probability',
ylabel='Lyapunov Exponent', color=COLORS['BW'], **fsaver('ly_pe_vars'))
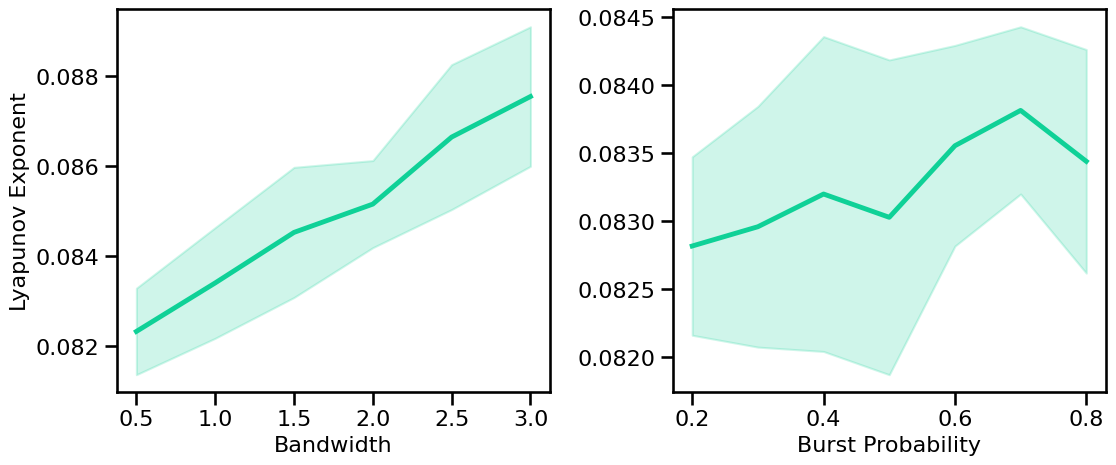
Conclusions¶
Lyapunov Exponent:
decreases with decreasing aperiodic exponent, non-linearly
increases to a point, then decreases, with increases oscillation frequency, non-linearly
increases with increasing oscillation power, non-linearly