Time Series Simulations
Contents
Time Series Simulations¶
This notebook introduces the time domain simulations used in this project.
Time series are simulated using neurodsp.
# Setup notebook state
from nbutils import setup_notebook; setup_notebook()
from neurodsp.sim import (sim_powerlaw, sim_synaptic_current, sim_knee,
sim_oscillation, sim_combined, sim_peak_oscillation)
from neurodsp.utils import set_random_seed
from neurodsp.plts import plot_time_series, plot_timeseries_and_spectra
from neurodsp.plts.utils import make_axes
# Import custom project code
from apm.io import APMDB
from apm.plts.utils import figsaver
from apm.sim.examples import get_times, get_examples
Settings¶
First, we will define some settings for the simulations.
# General simulation Settings
n_seconds = 10
fs = 500
# Component parameters
default_exp = -1.0
default_exp2 = -2.0
default_knee = 500
default_freq = 10
default_bw = 1.5
default_height = 1.5
f_range = (1, None)
# Collect together parameters for combined signals
comps_osc = {'sim_powerlaw' : {'exponent' : default_exp},
'sim_oscillation' : {'freq' : default_freq}}
comps_burst = {'sim_powerlaw' : {'exponent' : default_exp},
'sim_bursty_oscillation' : {'freq' : default_freq}}
peak_params = {'freq' : default_freq, 'bw' : default_bw, 'height' : default_height}
# Plot settings
plt_kwargs = {'xlabel' : '', 'ylabel' : ''}
# Settings for saving figures
SAVE_FIG = True
FIGPATH = APMDB().figs_path / '11_ts_sims'
# Create helper function to manage figsaver settings
fsaver = figsaver(SAVE_FIG, FIGPATH)
# Set random seed
set_random_seed(111)
Powerlaw Signal¶
First, we can simulate a ‘powerlaw’ signal, a 1/f signal that follow a powerlaw in the frequency domain.
# Simulate a powerlaw signal
sig_pow = sim_powerlaw(n_seconds, fs, default_exp, f_range=f_range)
# Plot simulated powerlaw signal and associated power spectrum
plot_timeseries_and_spectra(sig_pow, fs, **fsaver('powerlaw'))
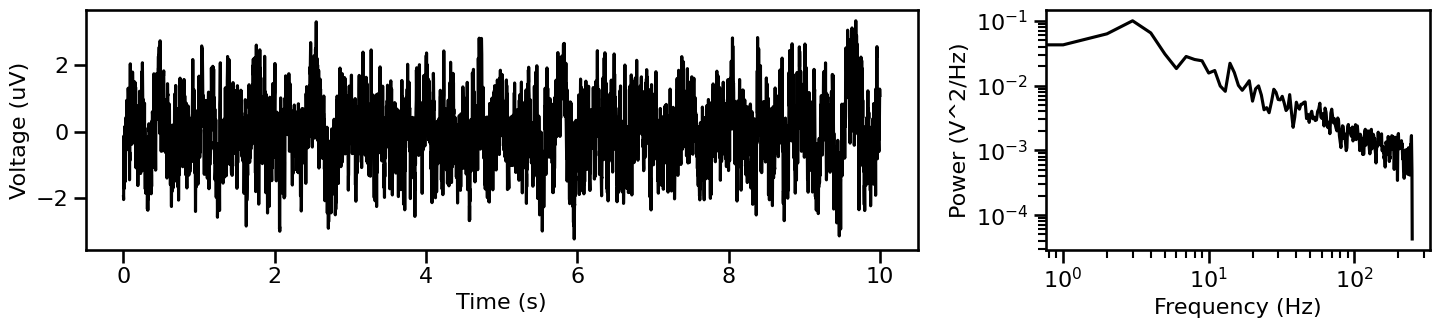
Continuous Oscillation¶
Next, we can simulate an oscillation, in this case, a continuous sinusoid.
# Simulate an oscillation
sig_osc = sim_oscillation(n_seconds, fs, freq=default_freq)
# Plot simulated oscillatory signal and associated power spectrum
plot_timeseries_and_spectra(sig_osc, fs, **fsaver('oscillation'))
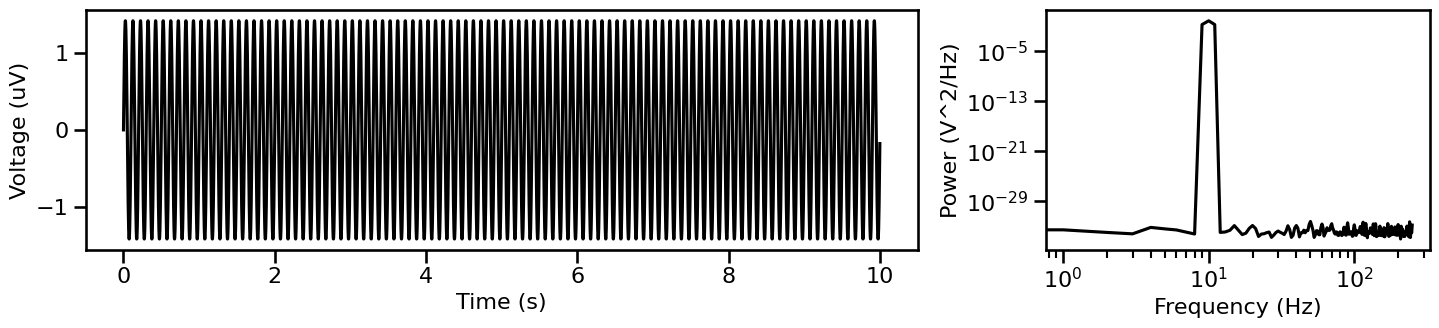
Combined Signal¶
Now we can can combine the aperiodic and periodic components from above, and create a combined signal.
# Simulate a combined signal, with an aperiodic and a periodic component
sig_comb = sim_combined(n_seconds, fs, comps_osc)
# Plot simulated combined and associated power spectrum
plot_timeseries_and_spectra(sig_comb, fs, **fsaver('combined'))
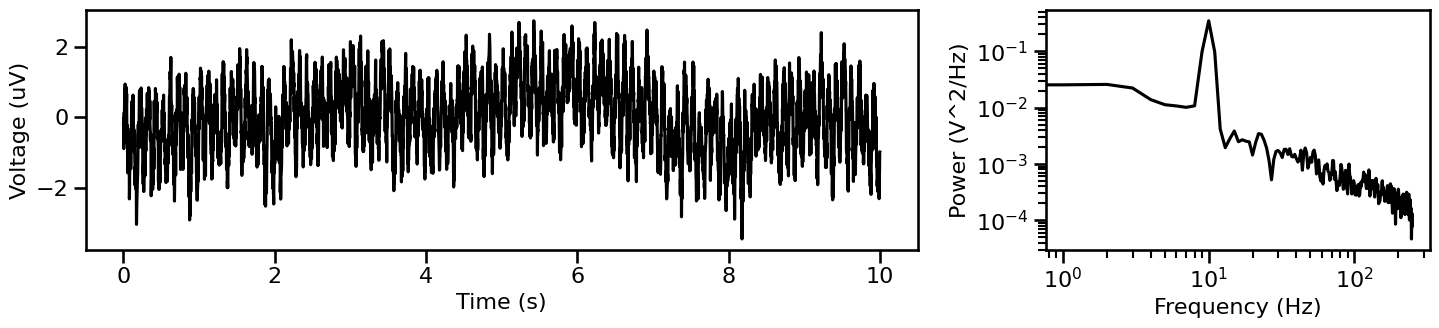
Synpatic Signal: Aperiodic Activity with a Knee¶
There are different possible ways to simulate aperiodic activity.
In this next simulation, we will simulate a ‘synpatic current’ model, which creates aperiodic activity with a knee.
# Simulate aperiodic activity from a synaptic current model
sig_syn = sim_synaptic_current(n_seconds, fs)
# Plot simulated synaptic signal and associated power spectrum
plot_timeseries_and_spectra(sig_syn, fs, **fsaver('syn_current'))
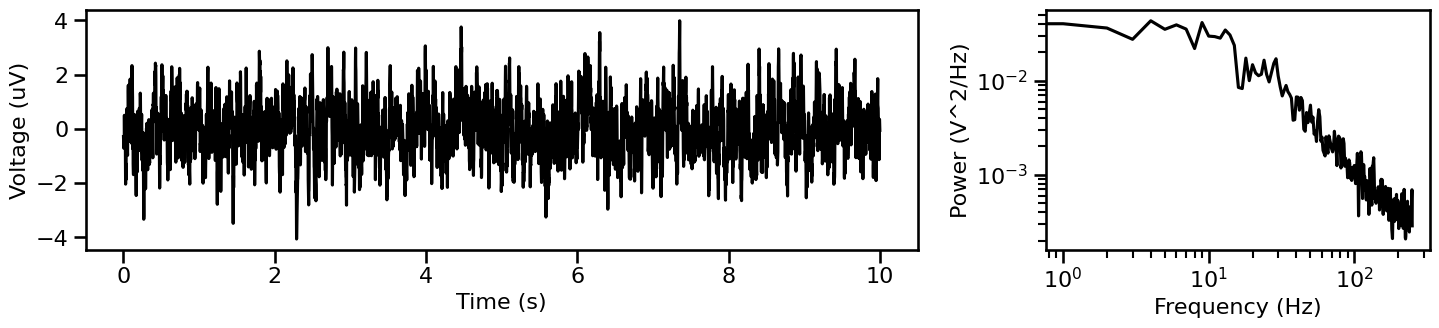
Aperiodic Signal with a Knee¶
We can also simulate signals with a knee without using ane explicit synaptic model.
# Simulate aperiodic activity from a synaptic current model
sig_knee = sim_knee(n_seconds, fs, 0, default_exp2, default_knee)
# Plot simulated knee signal and associated power spectrum
plot_timeseries_and_spectra(sig_knee, fs, **fsaver('knee'))
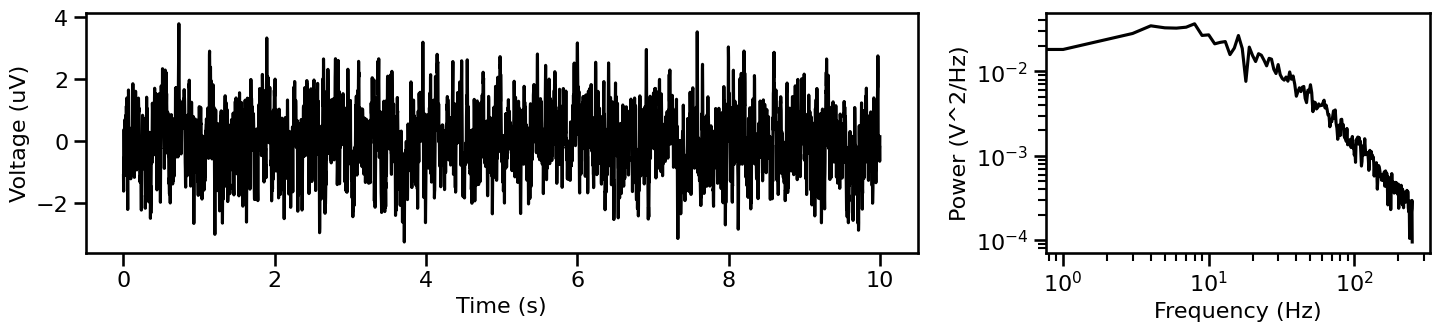
Bursty Oscillation¶
Oscillations are also not necessarily continuous. We we will simulate a combined signal with a bursty oscillation.
# Simulate a combined signal with a bursty oscillation
sig_burst = sim_combined(n_seconds, fs, comps_burst)
# Plot simulated bursty oscillatory signal and associated power spectrum
plot_timeseries_and_spectra(sig_burst, fs, **fsaver('burst_osc'))
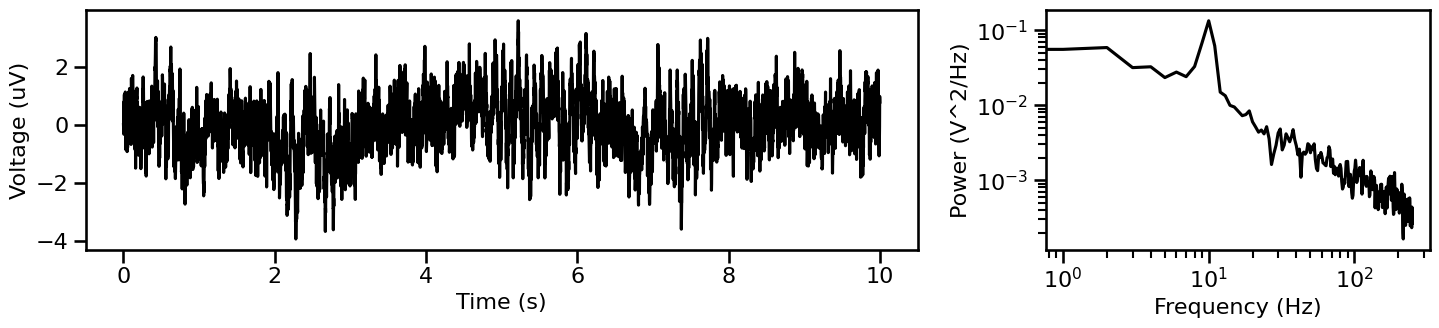
Peak Signal¶
Another dimension that signals can vary in is the bandwidth of a peak. Here, we will create a simulation in which we can specify the bandwidth of an oscillatory peak, that is simulated on top of an aperiodic component.
# Simulate a signal with a peak of a defined bandwidth
sig_peak = sim_peak_oscillation(sig_pow, fs, **peak_params)
# Plot simulated peak signal and associated power spectrum
plot_timeseries_and_spectra(sig_peak, fs, **fsaver('peak'))
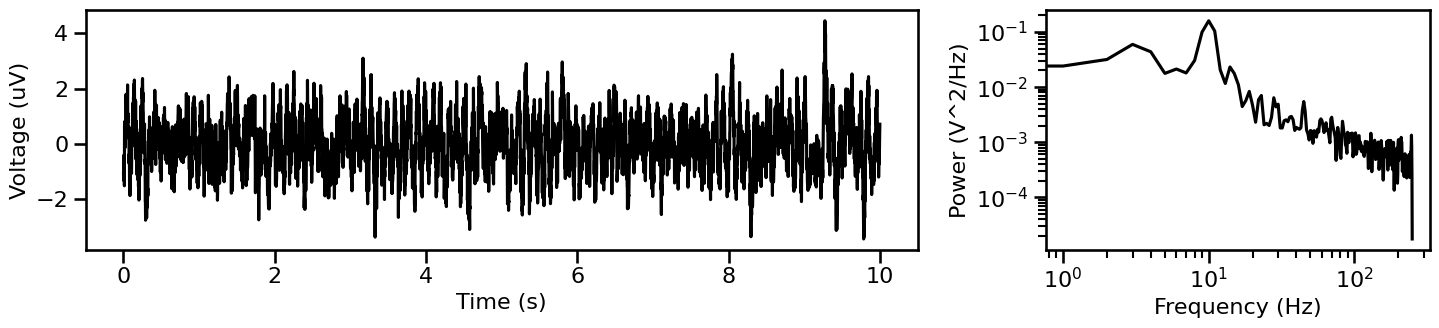
Example Time Series¶
In the notebooks that follow, you will see that measures are applied to a set of ‘example’ time series.
These examples are time series that have been pre-computed, using the functions above.
This set of example signals is shown here.
# Get example signals
times = get_times()
examples = get_examples()
# Plot example signals across different kinds of simulations
axes = make_axes(len(examples), 1, figsize=(10, 2 * len(examples)))
for ind, (label, sig) in enumerate(examples.items()):
plot_time_series(times, examples[label], title=label, xlim=[5, 10], **plt_kwargs, ax=axes[ind])
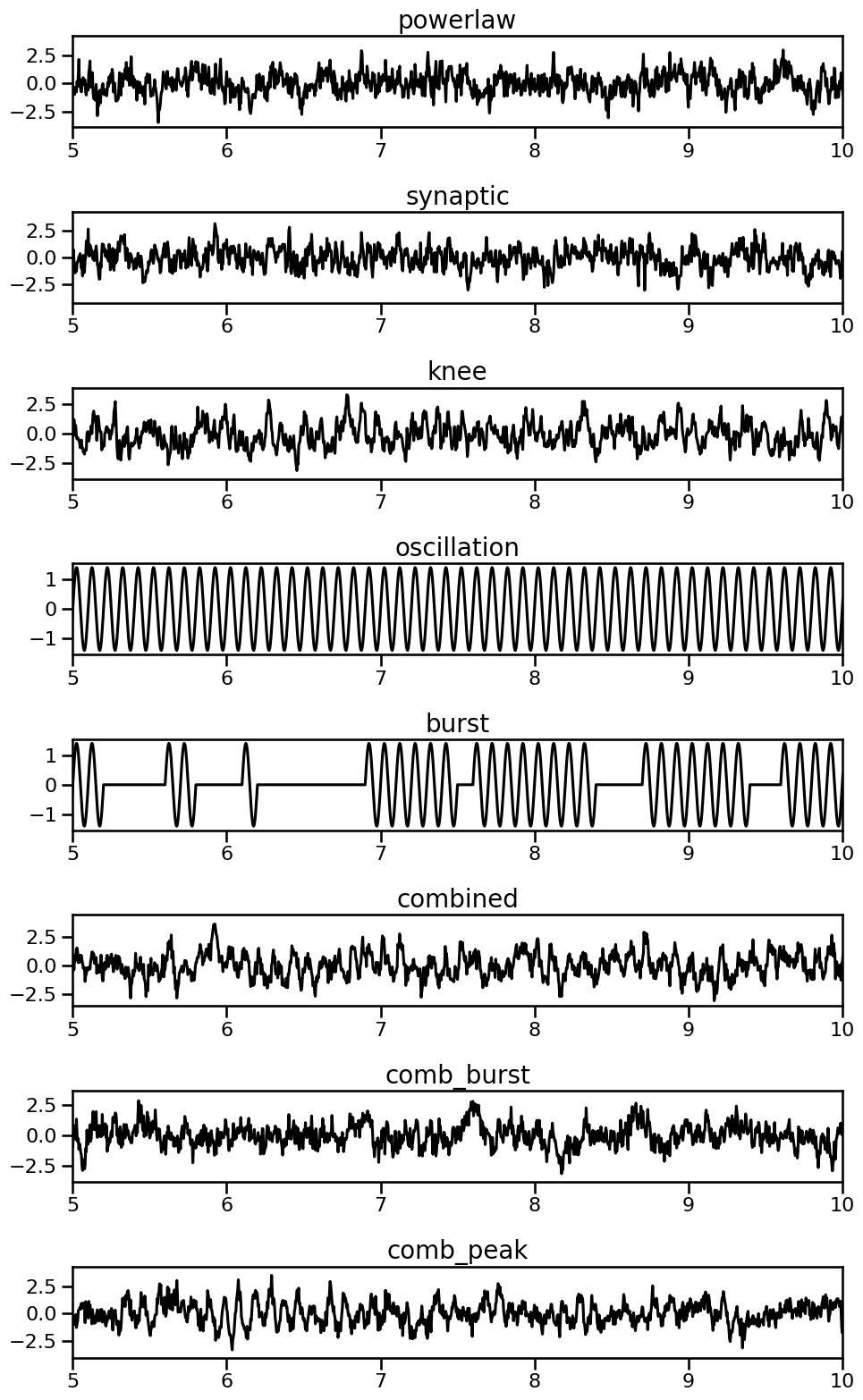